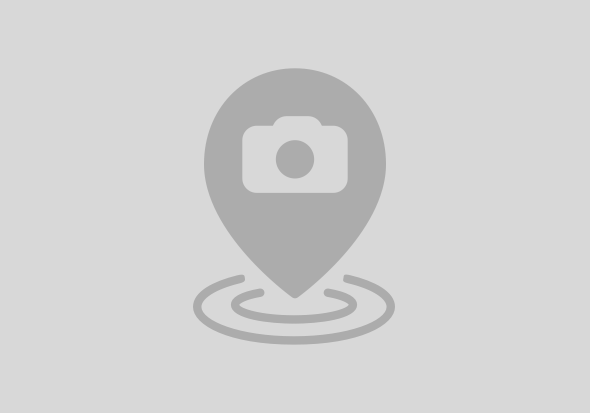
Hi All,
As part of one POC, we had a requirement to send SMS from ABAP. The obvious fact is that we need an SMS Gateway service provider to trigger SMS. Since it was just a POC so we were looking for some free or at least very cheap way to do it. After spending some time on Google, I found an easy and free way to achieve this functionality. The idea is to use your android device as SMS Gateway and consume REST API to trigger messages. 'SMSGateway.me' (https://smsgateway.me/) provides the exact functionality that too free of cost.
1. Install 'SMS Gateway Me' application on an Android phone. You can find it here SMS Gateway API – Android-Apps auf Google Play or at provider's website SMS Gateway - Android - Programmatically send messages
2. Till the time application gets installed, you can register on their website and create a free account. Otherwise you can also sign up for the new account from the app as well. Once you are registered, login to the device. After login, a device ID would be generated and device will start polling their server to fetch any pending SMS send requests.
3. Read the API documentation provided here https://smsgateway.me/sms-api-documentation/getting-started
4. Trigger a HTTP POST call to their messaging API.
5. Next time your device polls the server, it will fetch the SMS request that you have sent and will send the SMS to the mentioned number.
Steps 1 & 2 do not need an explanation.
3. Once you are on the API Documentation page, navigate to the message API section > Send message to number. The API details are as below:
(image source: https://smsgateway.me/sms-api-documentation/messages/send-message-to-number)
4. Now we have to trigger an HTTP POST call from ABAP code to the respective endpoint i.e. http://smsgateway.me/api/v3/messages/send with some mandatory parameters. You can find device ID from your mobile application which would look like:
Now we will create a FM to test this functionality. The FM could look something like below:
FUNCTION zsend_sms.
*"----------------------------------------------------------------------
*"*"Local Interface:
*" IMPORTING
*" VALUE(LV_EMP_MOBILE_NO) TYPE AD_TLNMBR
*" VALUE(LV_MSG) TYPE STRING
*" TABLES
*" RETURN STRUCTURE BAPIRET2 OPTIONAL
*"----------------------------------------------------------------------
DATA: lv_url TYPE string,
lo_http_client TYPE REF TO if_http_client,
lv_body TYPE string,
lv_result TYPE string,
c_email TYPE string,
c_password TYPE string,
c_deviceid TYPE string.
lv_url = 'http://smsgateway.me/api/v3/messages/send'.
c_email = 'youremail@example.com'.
c_password = 'MyPassword'.
c_deviceid = 'XXXXX'.
CALL METHOD cl_http_client=>create_by_destination
EXPORTING
destination = 'SMSGATEWAY_ME'
IMPORTING
client = lo_http_client
* EXCEPTIONS
* argument_not_found = 1
* destination_not_found = 2
* destination_no_authority = 3
* plugin_not_active = 4
* internal_error = 5
* others = 6
.
IF sy-subrc <> 0.
* Implement suitable error handling here
ENDIF.
CALL METHOD lo_http_client->request->set_method
EXPORTING
method = lo_http_client->request->co_request_method_post.
CALL METHOD lo_http_client->request->set_content_type
EXPORTING
content_type = 'application/json'.
CONCATENATE '{ '
'"email": ' '"' c_email '",'
'"password": ' '"' c_password '",'
'"device": ' '"' c_deviceid '",'
'"number": ' '"' lv_emp_mobile_no '",'
'"message": ' '"' lv_msg '"'
' }'
INTO lv_body.
call method lo_http_client->request->set_cdata
exporting
data = lv_body.
lo_http_client->send(
EXCEPTIONS
http_communication_failure = 1
http_invalid_state = 2 ).
lo_http_client->receive(
EXCEPTIONS
http_communication_failure = 1
http_invalid_state = 2
http_processing_failed = 3 ).
lv_result = lo_http_client->response->get_cdata( ).
ENDFUNCTION.
Thus we would receive the response from the server in lv_result which we can parse and process as per our needs.
Since your device is already polling the server, it will fetch whatever message you have queued and would send from the device. You can change the polling frequency from app settings.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
5 | |
3 | |
3 | |
2 | |
2 | |
2 | |
2 | |
2 | |
1 | |
1 |