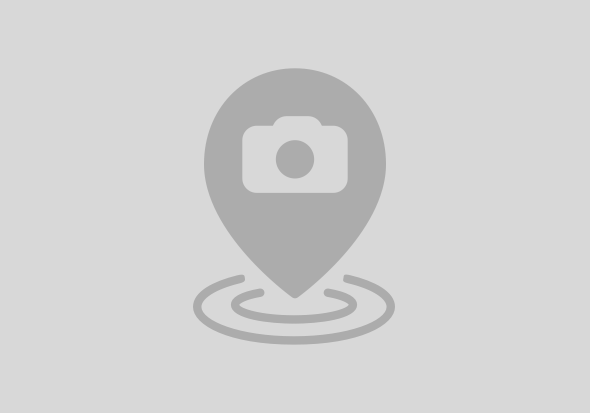
Purpose
if you try to allow end users to change the display setting for measures, try out this dialog template. It changes:
It works in any application, connected to DS_1 data source. This time no SDK, just a use of scripting in standard.
Important: you can connect this to a data source with different names, but you would need to catch all places where DS_1 is used and replace.
User Interface
This is how it looks like below.
Background
Many options can be changed by using scripting. This dialog is mainly using some script functions to
Framework
This implementation is NON-SDK, this is a simple composition of UI elements and scripts based on default functionality which is in standard. For the dialog I have used the way posted in my other document Design Studio Application with Custom Dialog where you can check details on dialogs.
It is as usual produced with release 1.3 SP1.
Workflow
The entry point is the hidden button "DIALOG_MEASURE_OPEN", there you can check up the onClick() method. Inline comments should give you the idea how it is created.
// first set the values you want to edit in the dialog...
// read all dimensions
var dimenstions = DS_1.getDimensions();
var measureDimensionId = "";
dimenstions.forEach(function(dimension, index) {
// find key figures (1.3 solution, language dependent; in 1.4 will bring better function "getMeasureDimension()")
// you need to change for other languages and add your language description for key figures
if(dimension.text == "Key Figures" || dimension.text == "-your-lang-description-" ) {
measureDimensionId = dimension.name;
}
});
// read first 100 members (should be ok for majority of queries)
var measureDimensionMembers = DS_1.getMembers(measureDimensionId, 100);
// initialize the dropdowns, can be one once, but the code here is not performance critical
DIALOG_MEASURE_DROPDOWN_MEASURES.removeAllItems();
// add initial entry, required to trigger "selected" event, could be changed to auto-select the first measure
DIALOG_MEASURE_DROPDOWN_MEASURES.addItem("N/A", " -- select measure --");
// add all measures
measureDimensionMembers.forEach(function(member, index) {
DIALOG_MEASURE_DROPDOWN_MEASURES.addItem(member.internalKey, member.text);
});
// decimals initialisation
DIALOG_MEASURE_DROPDOWN_DECIMALS.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_DECIMALS.removeAllItems();
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("DEFAULT", "Query Default");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("0", "0 (0)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("1", "0.0 (1)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("2", "0.00 (2)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("3", "0.000 (3)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("4", "0.0000 (4)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("5", "0.00000 (5)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("6", "0.000000 (6)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("7", "0.0000000 (7)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("8", "0.00000000 (8)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("9", "0.000000000 (9)");
DIALOG_MEASURE_DROPDOWN_DECIMALS.addItem("10", "0.0000000000 (10)");
// scaling initialisation
DIALOG_MEASURE_DROPDOWN_SCALING.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_SCALING.removeAllItems();
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("DEFAULT", "Query Default");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("1", "1");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("2", "10");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("3", "100");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("4", "1.000");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("5", "10.000");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("6", "100.000");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("7", "1.000.000");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("8", "10.000.000");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("9", "100.000.000");
DIALOG_MEASURE_DROPDOWN_SCALING.addItem("10", "1.000.000.000");
// zero initialisation
DIALOG_MEASURE_DROPDOWN_ZERO.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_ZERO.removeAllItems();
DIALOG_MEASURE_DROPDOWN_ZERO.addItem("DEFAULT", "Query Default");
DIALOG_MEASURE_DROPDOWN_ZERO.addItem("EMPTY_CELL", "Empty Cell");
DIALOG_MEASURE_DROPDOWN_ZERO.addItem("WITHOUT_CURRENCY_UNIT", "Without Currency");
DIALOG_MEASURE_DROPDOWN_ZERO.addItem("CUSTOM", "Custom");
// negative initialisation
DIALOG_MEASURE_DROPDOWN_NEGATIVE.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_NEGATIVE.removeAllItems();
DIALOG_MEASURE_DROPDOWN_NEGATIVE.addItem("LEADING_MINUS", "Leading Minus: -56");
DIALOG_MEASURE_DROPDOWN_NEGATIVE.addItem("PARENTHESES", "Parentheses: (56)");
DIALOG_MEASURE_DROPDOWN_NEGATIVE.addItem("TRAILING_MINUS", "Trailing Minus: 56-");
// fill in the values which are coming from the query, those are not dependent on selected measure
var zeroDisplayValue = DS_1.getZeroDisplay();
var enabledCustom = false;
if(zeroDisplayValue == ZeroDisplay.DEFAULT) {
DIALOG_MEASURE_DROPDOWN_ZERO.setSelectedValue("DEFAULT");
}
if(zeroDisplayValue == ZeroDisplay.CUSTOM) {
DIALOG_MEASURE_DROPDOWN_ZERO.setSelectedValue("CUSTOM");
enabledCustom = true;
}
if(zeroDisplayValue == ZeroDisplay.EMPTY_CELL) {
DIALOG_MEASURE_DROPDOWN_ZERO.setSelectedValue("EMPTY_CELL");
}
if(zeroDisplayValue == ZeroDisplay.WITHOUT_CURRENCY_UNIT) {
DIALOG_MEASURE_DROPDOWN_ZERO.setSelectedValue("WITHOUT_CURRENCY_UNIT");
}
var negativeNumberDisplayValue = DS_1.getNegativeNumberDisplay();
if(negativeNumberDisplayValue == NegativeNumberDisplay.LEADING_MINUS) {
DIALOG_MEASURE_DROPDOWN_NEGATIVE.setSelectedValue("LEADING_MINUS");
}
if(negativeNumberDisplayValue == NegativeNumberDisplay.PARENTHESES) {
DIALOG_MEASURE_DROPDOWN_NEGATIVE.setSelectedValue("PARENTHESES");
}
if(negativeNumberDisplayValue == NegativeNumberDisplay.TRAILING_MINUS) {
DIALOG_MEASURE_DROPDOWN_NEGATIVE.setSelectedValue("TRAILING_MINUS");
}
// initialize the ui elements in dialog
DIALOG_MEASURE_PREVIEW.setVisible(true);
DIALOG_MEASURE_CHECKBOX_APPLY.setChecked(false);
// show the background (styled for "blocking UI")
DIALOG_BACKGROUND.setVisible(true);
// show the dilaog frame
DIALOG_MEASURE.setVisible(true);
Then, the detailed changes are implemented in the onSelect() event in the dropdown "DIALOG_MEASURE_DROPDOWN_MEASURES", here the code
var selectedMeasure = DIALOG_MEASURE_DROPDOWN_MEASURES.getSelectedValue();
// apply change
if(selectedMeasure == "N/A") {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_SCALING.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_ZERO.setEnabled(false);
DIALOG_MEASURE_DROPDOWN_NEGATIVE.setEnabled(false);
DIALOG_MEASURE_DESC.setText("Select Measure...");
} else {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setEnabled(true);
DIALOG_MEASURE_DROPDOWN_SCALING.setEnabled(true);
DIALOG_MEASURE_DROPDOWN_ZERO.setEnabled(true);
DIALOG_MEASURE_DROPDOWN_NEGATIVE.setEnabled(true);
var measureDescription = DIALOG_MEASURE_DROPDOWN_MEASURES.getSelectedText();
DIALOG_MEASURE_DESC.setText("Define the display format for " +
measureDescription);
var scalling = DS_1.getScalingFactor(selectedMeasure);
if(scalling == Scaling.FACTOR_DEFAULT) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("DEFAULT");
}
if(scalling == Scaling.FACTOR_1) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("1");
}
if(scalling == Scaling.FACTOR_10) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("2");
}
if(scalling == Scaling.FACTOR_100) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("3");
}
if(scalling == Scaling.FACTOR_1000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("4");
}
if(scalling == Scaling.FACTOR_10000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("5");
}
if(scalling == Scaling.FACTOR_100000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("6");
}
if(scalling == Scaling.FACTOR_1000000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("7");
}
if(scalling == Scaling.FACTOR_10000000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("8");
}
if(scalling == Scaling.FACTOR_100000000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("9");
}
if(scalling == Scaling.FACTOR_1000000000) {
DIALOG_MEASURE_DROPDOWN_SCALING.setSelectedValue("10");
}
var decimal = DS_1.getDecimalPlaces(selectedMeasure);
if(decimal == -1) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("DEFAULT");
}
if(decimal == 0) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("0");
}
if(decimal == 1) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("1");
}
if(decimal == 2) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("2");
}
if(decimal == 3) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("3");
}
if(decimal == 4) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("4");
}
if(decimal == 5) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("5");
}
if(decimal == 6) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("6");
}
if(decimal == 7) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("7");
}
if(decimal == 😎 {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("8");
}
if(decimal == 9) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("9");
}
if(decimal == 10) {
DIALOG_MEASURE_DROPDOWN_DECIMALS.setSelectedValue("10");
}
// prepare current value and preview
var preview = DS_1.getDataAsString(selectedMeasure, {});
if(preview == "") {
preview = "Measure Not in Resultset";
DIALOG_MEASURE_CURRENT.setText(preview);
DIALOG_MEASURE_PREVIEW.setText("");
} else {
if(preview.indexOf("-") == -1 && preview.indexOf("(") == -1) {
var selectedNegative = DIALOG_MEASURE_DROPDOWN_NEGATIVE.getSelectedValue();
if(selectedNegative == "LEADING_MINUS") {
preview = "-" + preview;
}
if(selectedNegative == "PARENTHESES") {
preview = "(" + preview + ")";
}
if(selectedNegative == "TRAILING_MINUS") {
preview = preview + "-";
}
}
DIALOG_MEASURE_CURRENT.setText(preview);
DIALOG_MEASURE_PREVIEW.setText(preview);
}
}
the apply functions for the real changes are implemented as onSelect() event in the corresponding dropdowns. To save code, those are also called when OK button is pressed.
Use
You can simply also use this dialog and copy and paste it into the "ADHOC" Application created from the delivered application template. Just copy it to the end and attach from any image / button even (if someone needs help on this I can make this more concrete).
When coping, do not forget to transwer also the CSS styles.
This is how it should look like in the outline:
Source Code & Application
All available in local mode on GitHub:
For better download I have created a release of this repository here:
Question / Opinions?
now the content is open for discussion.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
18 | |
16 | |
12 | |
11 | |
9 | |
8 | |
8 | |
8 | |
7 | |
7 |