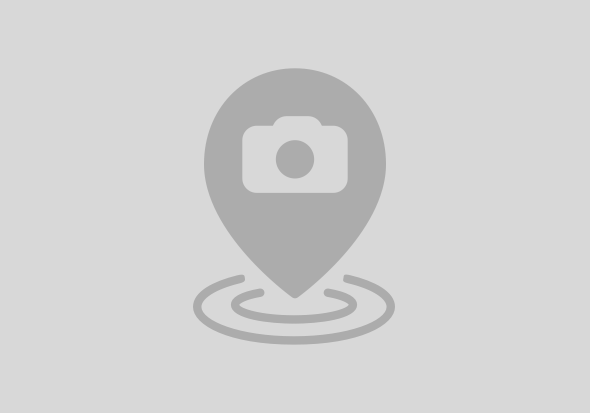
Intro
I would like to show you how to create a WebSocket chat application to send and receive a WhatsApp message from the SAPUI5 web browser with the open source Yowsup.
How it works?
Firstly, client will establish the connection to the server via the websocket address: ws://localhost:8080/fd6/websocket and register the following event listeners:
onerror: When a WebSocket encounters a problem, the "onerror" event handler is called.
onopen: When a WebSocket in the open state, the "onopen" event handler is called.
onmessage: When a WebSocket receives new data from the server, the "onmessage" event handler is called.
var webSocket = new WebSocket('ws://localhost:8080/fd6/websocket');
webSocket.onerror = function(event) {
onError(event)
};
webSocket.onopen = function(event) {
onOpen(event)
};
webSocket.onmessage = function(event) {
onMessage(event)
};
Once the connection to the server is established, it can send and receive the WhatsApp message with the following steps:
Send Message to WhatsApp
Step 1
When user sends a message from the browser, the function sendMsg() will send the message to the server: webSocket.send(msg);
function sendMsg() {
var msg = oMsg.getValue();
if (msg == "")
notify('Info', 'Please enter a message', 'information');
else {
webSocket.send(msg);
oMsg.setValue();
oMsg.focus();
lastMsg = oModel.oData.chat;
if (lastMsg.length > 0)
lastMsg += "\r\n";
oModel.setData({
chat : lastMsg + "You:" + msg
}, true);
$('#chatBox').scrollTop($('#chatBox')[0].scrollHeight);
}
return false;
}
Step 2
Method goInteractive in the python Yowsup code listens for the incoming message from the server: message = self.ws.recv() and send the message to the WhatsApp: msgId = self.methodsInterface.call("message_send", (jid, message))
def goInteractive(self, jid):
print("Starting Interactive chat with %s" % jid)
jid = "%s@s.whatsapp.net" % jid
print(self.getPrompt())
while True:
message = self.ws.recv()
if not len(message):
continue
if not self.runCommand(message.strip()):
msgId = self.methodsInterface.call("message_send", (jid, message))
self.sentCache[msgId] = [int(time.time()), message]
self.done = True
Receive Message from WhatsApp
Step 3
If there is any incoming message from WhatsApp, method onMessageReceived is called. It will send the message to the server: self.ws.send(messageContent)
def onMessageReceived(self, messageId, jid, messageContent, timestamp, wantsReceipt, pushName, isBroadcast):
if jid[:jid.index('@')] != self.phoneNumber:
return
formattedDate = datetime.datetime.fromtimestamp(timestamp).strftime('%d-%m-%Y %H:%M')
print("%s [%s]:%s"%(jid, formattedDate, messageContent))
if wantsReceipt and self.sendReceipts:
self.methodsInterface.call("message_ack", (jid, messageId))
print(self.getPrompt())
self.ws.send(messageContent)
Step 4
Finally, the function onMessage will print out the incoming message from the server to the client browser.
function onMessage(event) {
msg = event.data
lastMsg = oModel.oData.chat;
if (lastMsg.length > 0)
lastMsg += "\r\n";
oModel.setData({
chat : lastMsg + "WhatsApp:" + msg
}, true);
$('#chatBox').scrollTop($('#chatBox')[0].scrollHeight);
notify('WhatsApp', msg, 'information');
}
To display the message, I am using noty - a jQuery Notification Plugin:
function notify(title, text, type) {
// [alert|success|error|warning|information|confirm]
noty({
text : text,
template : '<div class="noty_message"><b>'
+ title
+ ':</b> <span class="noty_text"></span><div class="noty_close"></div></div>',
layout : "center",
type : type,
timeout : 4000
});
}
It's pretty easy to use and implement the WebSocket in SAPUI5. Thanks for reading my blog and let me know if you have any comments/questions. Enjoy chatting !
Source Code:
I have attached the complete source code fd6.war (rename fd6.war.txt to fd6.war) and the modified Yowsup python code in the GitHub:
ferrygun/WebSocketChat · GitHub
References:
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
7 | |
5 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |