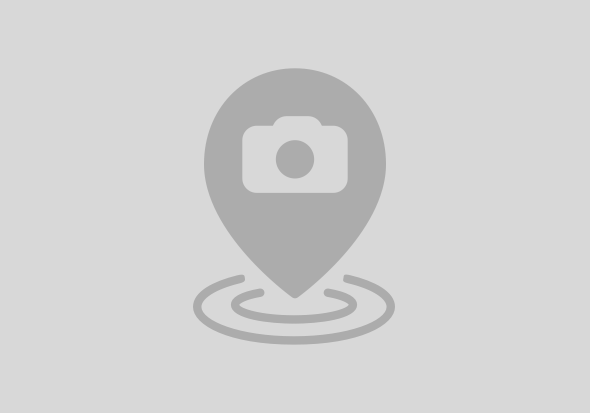
To create a simple application, use template "SAPUI5 Application" in WebIde.
This step will create the structure and files to have a simple SAPUI5 application.
To init model, several ways are possible.
In this example I create a very simple local json file name data.json in folder model :
{
"firstname":"John",
"lastname":"Smith"
}
In component.js, create model :
var oJSONDataModel;
oJSONDataModel= new sap.ui.model.json.JSONModel("model/data.json");
this.setModel(oJSONDataModel);
In view, add two input fields binded to model:
<Input width="100%" id="__input0" value="{/firstname}"/>
<Input width="100%" id="__input1" value="{/lastname}"/>
Then add two buttons :
<Button text="Save" width="100px" id="__button0" press="handleSave"/>
<Button text="Read" width="100px" id="__button1" press="handleRead"/>
The first one will be used to save data from our form to local database, and the second one to read data from local database to our form.
Now, we will adapt controller to add functions to manage button actions.
For save button, the code below create DB & Object if needed and add data from model to local DB :
handleSave : function (evt) {
var oData = this.getView().getModel().oData; //get data from Model
var request = window.indexedDB.open("offline_db", 1); //Open DB
request.onupgradeneeded = function(event){ //Object Stores don't exist
var db = event.target.result;
var objectStore = db.createObjectStore("mydata"); //create object store
};
request.onsuccess = function(event){//Objet Stores exist
this.myDB = event.target.result;
// Write to local DB
var oTransaction = this.myDB.transaction(["mydata"], "readwrite");
var oDataStore = oTransaction.objectStore("mydata");
oDataStore.delete(1);
oDataStore.add(oData, 1); //1 is the key out-of-line
};
}
For read button, the code below will open the local DB & read object to get data saved in local DB.
Then update model with this data.
handleRead : function (evt) {
var oJSONDataModel;
var oView = this.getView();
var request = window.indexedDB.open("offline_db", 1); //Open DB
request.onsuccess = function(event){//Objet Stores exist
this.myDB = event.target.result;
//Read from local DB
var oTransaction = this.myDB.transaction(["mydata"], "readwrite");
var oDataStore = oTransaction.objectStore("mydata");
var items = [];
oDataStore.openCursor().onsuccess = function(event) {
var cursor = event.target.result;
if (cursor) {
items.push(cursor.value); //add value to items table
cursor.continue();
}
else { //All data in items
oJSONDataModel = new sap.ui.model.json.JSONModel();
oJSONDataModel.setData(items[0]); //Create model with local DB data
oView.setModel(oJSONDataModel); //Update model used by view
oView.getModel().refresh(true);
}};
};
}
Application is now ready to do first test !!
- Deploy & run application.
- Click on save button
- Open chrome dev tools and check in resources tab "Indexed DB". "offline_db" should be there and in mydata, you should see data.
- Now, change form data or clear them.
- Click on read button, form should be updated with data stored in local DB
Now, we can use local storage to save data but to be able to use application offline, we have to cache all needed files.
To cache application, we have use cache.manifest to list files to cache in browser.
Create a file cache.manifest.
This file looks like this one :
CACHE MANIFEST
#Version 0.15
CACHE:
/index.html
/css/style.css
/resources/sap/ui/core/themes/sap_bluecrystal/library.css
/resources/sap-ui-core.js
/resources/sap/ui/core/library-preload.json
/resources/sap/m/library-preload.json
/resources/sap/ui/core/themes/sap_bluecrystal/library.css
/resources/sap/m/themes/sap_bluecrystal/library.css
/resources/sap/ui/core/themes/sap_bluecrystal/library-parameters.json
/resources/sap/m/themes/sap_bluecrystal/library-parameters.json
/resources/sap/m/messagebundle_fr.properties
/Component-preload.js
/manifest.json
/i18n/i18n.properties
/resources/sap/ui/layout/library-preload.json
/resources/sap/ui/layout/themes/sap_bluecrystal/library.css
/resources/sap/ui/layout/themes/sap_bluecrystal/library-parameters.json
/resources/sap/ui/core/messagebundle_fr.properties
/model/data.json
NETWORK:
*
Now, add it in index.html file
<html manifest="/cache.manifest">
Our application will be now cached by browser and be able to be used offline.
Check it with devtools in network tab that files are loaded from cache (after the first load !):
Don't forget to change cache.manifest file when your update application to be sure that browser get the last version of files changed.
Below several useful links:
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |