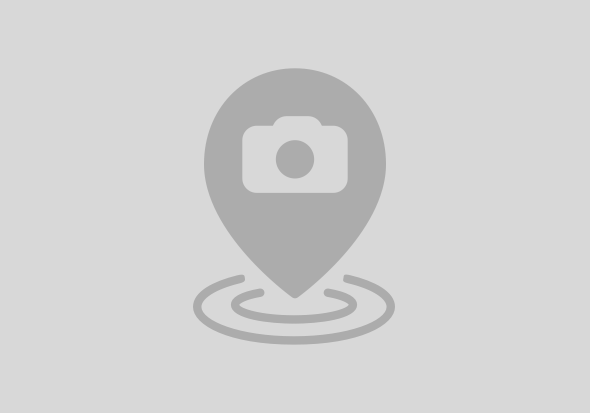
This blog is about Integration Gateway (IGW) in SAP Mobile Platform 3.0 (SMP).
The Integration Gateway component allows to expose backend data as an OData service, where some concrete data sources are supported more or less automatically
Since SMP SP09, a new data source has been introduced: the “Custom Code” data source, that gives the developer more freedom to access different data sources.
In the first 3 blogs, we’ve done some exercises to write some code that provides hard-coded dummy data.
In this current blog, I’d like to share another sample code, to showcase how Integration Gateway can be used to expose any data in a freestyle way.
It is just meant to give you another idea to play around with this feature, without using a complex backend data source.
In this example, we’re using some data that is present in the runtime environment, so we don’t have to care about connections and so on to receive data.
As a prerequisite, you should have checked the introduction to the “Custom Code” data source here.
For our example, we’ll create a simple scenario:
Our OData service will provide a list containing all request Headers that are accessible during runtime from the script.
Don’t ask for which purpose we do that 😉 it is just an example.
It’s just some data that is accessible from our project without additional effort.
And we offer a function import that returns the amount of all headers.
This function import could serve as a workaround while $count is not supported.
The OData model looks like this:
Bind data source
The Entity Set “HeaderSet” and the Function Import “getHeaderCount” are both bound to the data source “Custom Code”.
First, we’ll implement the getFeed() method in order to have a working OData service providing some payload.
We’ll ignore the other operations
Then we’ll implement the function import.
When the Integration Gateway component invokes our script, it passes some necessary information to us, including the headers.
Headers are used to carry information along with HTTP requests, therefore they are relevant for Integration Developers.
So the service we’re creating in this tutorial will make your dreams come true;-)
In our script, we access the headers as usual, then we put them in a map and provide them as list.
When invoking our OData service, we’ll be able to see all headers that are accessible in the “Custom Code” data source.
The code:
function getFeed(message){
importPackage(java.util);
importPackage(java.lang);
var responseList = new ArrayList();
// add the headers that are passed from the FWK
var headersMap = message.getHeaders();
addMapToList(headersMap, responseList);
// now add the headers that are sent by the user or by the browser
var odataContextHeader = headersMap.get("odatacontext");
if(odataContextHeader){
var outsideHeaders = odataContextHeader.getRequestHeaders();
addMapToList(outsideHeaders, responseList);
}
message.setBody(responseList);
return message;
}
function addMapToList(headersMap, responseList) {
if(! headersMap){
return;
}
var it = headersMap.keySet().iterator();
var id = responseList.size();
while (it.hasNext()) {
id++;
var headerKey = it.next();
var headerValue = headersMap.get(headerKey);
var headerType = null;
var headerValueString = null;
if(headerValue){
headerValueString = headerValue.toString();
headerType = headerValue.getClass().getCanonicalName();
}
// build the map for the OData entity
var responseMap = new HashMap();
responseMap.put("ID", Integer.toString(id));
responseMap.put("Name", headerKey);
responseMap.put("Value", headerValue);
responseMap.put("Type", headerType);
responseList.add(responseMap);
}
}
Note that the sample code contains 2 methods, the first one is the callback method that is generated by Eclipse, the second one is a helper method that is used by us.
The URL to invoke:
https://localhost:8083/gateway/odata/SAP/<MYSERVICE>/HeaderSet
Our implementation also takes user headers into account, this means you can use a REST client to invoke the HeaderSet (GET request) and specify some additional headers.
They will be added to the response payload of our service.
The function import that we’ve defined in our model can be invoked with a GET request and it will provide the total amount of accessible headers as an integer value in the payload of the response.
If the function import has a return type, the return value has to be set to the message object in the correct type.
In our simple example, we’ve designed the return type as Edm.int16, so we have to return an integer.
The code looks as follows:
function processFunctionImport(message){
importPackage(java.lang);
// the FWK headers
var headersMap = message.getHeaders()
var size1 = headersMap.size();
// add the user headers
var size2 = 0;
var odataContextHeader = headersMap.get("odatacontext");
if(odataContextHeader){
size2 = odataContextHeader.getRequestHeaders().size();
}
message.setBody(new Integer(size1 + size2));
return message;
}
The URL to invoke:
https://localhost:8083/gateway/odata/SAP/<MYSERVICE>/getHeaderCount
Again, you can use a REST client to invoke the Function Import and specify some additional headers, to see how the count result reacts to it.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
41 | |
25 | |
17 | |
14 | |
9 | |
7 | |
6 | |
6 | |
6 | |
6 |