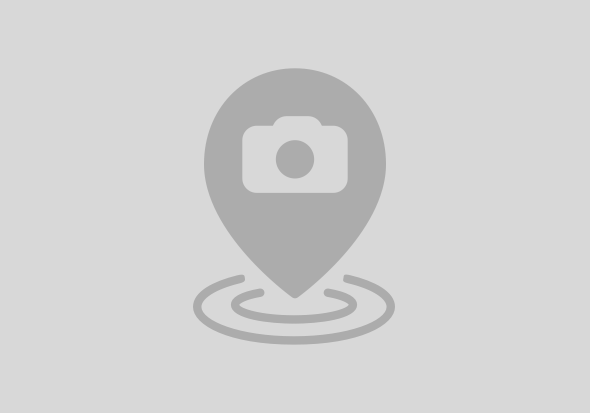
Hello Everyone,
I recently had to create some demo’s to visualize data form a HANA XS system. The team created some
odata (xsodata) services for me and “the asks” was to have a UI5/Fiori App to display the data via different charts.
There are lot of example and posts out there but I could not find answers to some of the issue I was facing hints this post.
Here the resulting application which we built using UI5.
The app basically displays sales information from one odata service in different ways.
Here are some of the challenge I was facing during prototyping:
1. CORS (http://en.wikipedia.org/wiki/Cross-origin_resource_sharing)
.
Since I started my development locally using JSbin I kept running into the CORS issue.
One simple work around is to use chrome and start
via the window->run or dos prompt using the parameters like:
chrome.exe --disable-web-security or
chrome.exe --disable-web-security --allow-file-access-from-files
to allow local file access.
Note: Make sure you close all chrome.exe task/sessions before starting chrome with the command line parameters.
You should see a message in the chrome header like:
Here an example of an SAPUI5 chart which you can run the code locally with a local json source
(http://jsbin.com/cikudo/5/edit?html,output) or use the attached zip files or Github url listed at the end of the post.
If the browser security is set properly and the json data file is I the right path you should see the following
picture after executing the jsbin example.
2. Differences between xml and json model from a odata service.
One of the challenges I ran into was that there are different ways to bind the data from odata service using xml or
json format. The normal odata service call will invoke $metadata and basically requires a working real odata service vs
json you can work with offline json files like in my jsbin examples or attatched zip file/github.
In order to bind odata you just need to used the entity url path.
EXAMPLE:
path: "/Opportunities", // xsodata nativ binding (xml)
path: "/d/results", // Json xsodata binding for the same service
Note: The json model binding for xsodata service always uses the path "/d/results”.
Here an example of a bubble chart using odata (xml) model.
Code in JSBin http://jsbin.com/zifoki/4/edit
JSbin with the local json data is a great place to start “fiddling” with the data and the chart code but eventually you
want to move to odata to take advantage of all the odata functionality especially once the charts and data get more complex.
3. Which chart library to use?
SAP UI5 offers viz and makit and there several other libraries out there. I just focused on makit and viz and ended up using viz.
Here the SAP demo app for the makit library.
https://sapui5.netweaver.ondemand.com/sdk/test-resources/sap/makit/demokit/index.html
Here the SAP demos for the viz library
https://sapui5.hana.ondemand.com/sdk/test-resources/sap/viz/Charting.html
Here the code for a json makit Pie chart example Example: http://jsbin.com/niqed/3/edit
There was no particular reason why I ended up with viz over the makit library other than there seems more
information on sap.viz out there. There also other chart libraries available like d3.js, c3.js and pivot.js which
can be used see alternative libraries section below for examples.
4. Layouts
One of the challenges was to layout 6 charts (see 1st picture) on one page and make sure its visible on an ipad. Some of the charts do
not seem to display when just adding them to a page like with .placeAt and some do show right away. A simple work around seems
to be to add the chart first to a panel and than place it on the page. Sometimes changing the height to pixel vs % worked too.
Example of using panel to place charts on a page.
Starting with a Panel layout to build the prototype worked great. We use matrix layout later to be a more
sophisticated.
EXAMPLE of Panel layout
var oPanel1 = new
sap.ui.commons.Panel({width: "100%",height: "100%"});
oPanel1.addContent(oChart);
oPanel1.setTitle(new sap.ui.core.Title({text: "Sale at Risk by Region"}));
oPanel1.placeAt("oPanel1");
The key was to add the some of the charts/elements to a cell before adding the layout to the page.
EXAMPLE of a Matrix layout
var oLayout = new sap.ui.commons.layout.MatrixLayout("layout", { width : "100%", columns : 2 });
var oCell = new sap.ui.commons.layout.MatrixLayoutCell({id : 'Cell-0-0',lSpan : 2 });
oCell.addContent(vbar);
oLayout.createRow(oCell);
var oCell10 = new sap.ui.commons.layout.MatrixLayoutCell({id : 'Cell-1-0',colSpan : 1 });
oCell10.addContent(oTable);
oLayout.createRow(oChart2,mycol2);
oLayout.placeAt("content");
Result of adding a Table to matrix layout cell.
Here a jsbin play ground of different charts and layouts: http://output.jsbin.com/zaropi/30
5. Aggregation of data.
Once I got more comfortable with the charts and the data model. I tried create the page and charts based on
the requirements. It took me a while till I realized that the charts never showed the sum of any of the values
e.g. Sales by region instead it show just the last customers of the region for example.
Example of a chart without aggregated and with aggregated data values form the same service.
It took a while to find the solution since the issue it was in the service it self and just partiality in the code.
The HANA xsodata service had a Vidal parameter missing called “aggregates always;” without that parameter
the service would not a aggregate values.
here the xsodata service In Hana Studio with the right parameter.
Below a Odata selection url to check the aggregation of values: format: <service/Entity url>?$parameter&$otherodataparameter
…/sapphire/opportunityforecast.xsodata/Opportunities/?$select=OPPORTUNITY_VALUE,CUSTOMER_DESC&$filter=startswith(CUSTOMER_DESC,'A')
Result without the aggregate parameter in HANA service
<d:CUSTOMER_DESC m:type="Edm.String">ABC Motorsports</d:CUSTOMER_DESC>
<d:OPPORTUNITY_VALUE m:type="Edm.Double">1125</d:OPPORTUNITY_VALUE>
With the parameter it returns the sum/total of the value for ABC Motorsports
<d:CUSTOMER_DESC m:type="Edm.String">ABC Motorsports</d:CUSTOMER_DESC>
<d:OPPORTUNITY_VALUE m:type="Edm.Double">6837050</d:OPPORTUNITY_VALUE>
The other issue was that like in odata url also in UI5 we have to define/select the fields for the aggregration.
Like:
var oDataset = new sap.viz.ui5.data.FlattenedDataset({
dimensions: [{
axis: 1,
name: 'REGION',
value: '{REGION}'
}],
measures: [{
name: 'VALUE',
value: "{OPPORTUNITY_AMOUNT}"
data: {
path: "/Opportunities",
parameters: {select: 'CUSTOMER_ID,REGION,CUSTOMER_DESC,OPPORTUNITY_AMOUNT'}
}
});
Otherwise the data would not be aggregated even if the service is setup right.
Here the Jsbin code example: http://jsbin.com/cotuso/4/edit
6. Filters and Sorters.
In order to filter and sort the data in the same way you can do this via odata url parameters
(http://www.odata.org/documentation/odata-version-3-0/url-conventions/) you need to add sorter and filters to the dataset.
Filter code example: (http://jsbin.com/cotuso/4/edit)
data: {
path: "/Opportunities",
filters: new sap.ui.model.Filter('VERSION',sap.ui.model.FilterOperator.EQ,'ACTUAL'),
sorter : new sap.ui.model.Sorter("REGION",true)
parameters: {select: 'CUSTOMER_ID,REGION,CUSTOMER_DESC,OPPORTUNITY_AMOUNT'} }
});
7. Alternative chart libraries
As mentioned before there are a lot of other chart JavaScript libraries out there which you can use instead of makit or viz.
D3 is a very popular one there are ui5 examples on sdn using d3 to render charts and gauges.
Here some other (code) example of charting libraries.
C3 - http://jsbin.com/vuhoy/1/edit?html,js,output
highcharts - http://www.highcharts.com/jsbin/#javascript,live
google charts - https://jsfiddle.net/api/post/library/pure/
The picture shows a d3 chart of the odata HANA service which I used in all my examples. You can find the code
for the above chart here. http://jsbin.com/vakiqa/6/edit?html,output
8. Adding you code to a Fiori Launchpad.
Here some quick infos on how to add you ui5 app to a Fiori Launchpad which was very useful.
http://scn.sap.com/community/mobile/blog/2014/05/04/how-to-setup-the-sap-fiori-Launchpad
Note: You can upload your developed code as a BSP page using se80 or the report/UI5/UI5_REPOSITORY_LOAD
… more information here
http://help.sap.com/saphelp_nw74/helpdata/en/91/f3ecc06f4d1014b6dd926db0e91070/frameset.htm
Conclusion:
There is of course a lot more to know about charts and ui5 data visualization but this will give you a good start and focus on the data, and charts
functionality and hopefully you avoid some of the pitfalls.
The code for all the examples is available in the jsbin, github and in the attached zip file.
Please leave a comment and let me know if you have questions or contact me via twitter
@markusvankempen or email me mvk@ca.ibm.com
Cheers
Markus
References:
Github: https://github.com/markusvankempen/SAPUI5-Charts
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 | |
3 | |
2 |