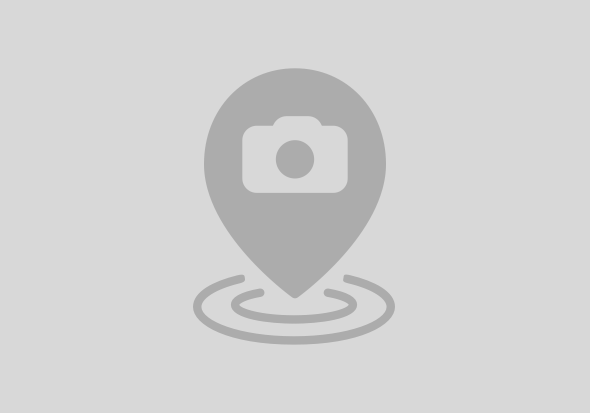
With the new and upcoming release of SAP BusinessObjects Business Intelligence Platform 4.2, you are no longer bound to either Java or .NET in order to manage your BI platform though the SDK.
The new BI Platform has an extended set of new API’s through the Business Intelligence Platform RESTful Web Services.
REST stands for Representational State Transfer, and is a way to communicate with another system though standard HTTP, and HTTP commands like GET, POST, DELETE ect.
The beauty about using the new REST API, is than you are no longer bound to use the old API’s which required JAVA or .NET language skills. This new API can be used with any other language that can use the HTTP protocol, so now you can use other languages like PERL, PHP, Node.js, PowerShell ect.
In this blogpost, I will show how easy it is to
And this all done in PowerShell :smile:
Before you can use the RESTful Web Service, you need to make sure it is up an running.
You can simply try to open and url like this http://<url of your BI platform>:6405/biprws
Port 6405 is the standard port for the BI Platform RESTful Web Service, and I recommend that you also take a look on the Business Intelligence platform RESTful Web Service Developer Guide 4.2 found on the Help SAP portal SAP BusinessObjects Business Intelligence platform 4.2 – SAP Help Portal Page
For this short demonstration, I have chosen to use the PowerShell comandlet Invoke-RestMethod, which is an easier to use than Invoke-WebRequest, but does not handle HTTP status codes and therefore I have “assumed” nothing fails in my code examples.
A good developer would most likely have added all sorts of error handling and checking :wink:
So let us get started
I have done my editing and testing in the PowerShell ISE, but you could as well just type it all in the PowerShell command window.
First I start by defining some variables I need to use for logon
# Define username and password used to logon
$userName = "Administrator"
$passWord = "Password1"
$authType = "secEnterprise" # can be either secEnterprise, secLDAP, secWinAD or secSAPR3
Second I define some variables for the ease of use, so if I change the baseURL, I do not need to go over all the lines of code
# Define the base URL and URLs for logon and logoff
$baseURL = "http://localhost:6405/biprws"
$logonURL = "$baseURL/logon/long"
$logoffURL = "$baseURL/logoff"
Then I create a generic dictionary to hold the HTTP headers needed for communicating with RESTful Web Service.
# Create a generic dictionary to hold the headers needed to communicate with the BI Rest Web Service
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Accept","Application/Json")
$headers.Add("Content-Type","Application/Json")
In my example I have chosen to use JSON as the content type. I could have used Application/xml instead, as the service supports that as well, but for my purpose it was easier to work with the JSON format.
Next I created the object needed to create a new user as JSON
$logonJson = @"
{
"userName": "$userName",
"password": "$passWord",
"auth": "$authType"
}
"@
Next I use the Invoke-RestMethod to logon on the BI platform and get the SAP Logon Token I need for further communication with the BI Platform
# Log on to the BI Platform
$response = Invoke-RestMethod -Uri $logonURL -Method Post -Headers $headers -Body $logonJson
# Retrieve the logonToken, this assumes everything went OK, since Invoke-RestMethod doesn't support .StatusCode
$SAPToken = $response.logonToken
And finally I add this token to the HTTP header collection, so I don’t have to code username and password into all the other requests
# Add the logonToken to the headers collection
$headers.Add("X-SAP-Logontoken",$SAPToken)
If I am not certain on how a command should look for creating a new user, and can allways call a GET request to /users/user to receive a template that can be used in the request body of a POST request to the same URL, like
$response = Invoke-RestMethod -Uri "${baseUrl}/users/user " -Method Get -Headers $headers
$response | ConvertTo-Json
Now when I have this template I can create the JSON used to create the new user
# Create json for a new user
$userJson = @"
{
"emailAddress": "",
"isPasswordToChangeAtNextLogon": false,
"isPasswordChangeAllowed": false,
"description": "This user was created by a script",
"fullName": "Demo User 01",
"newPassword": "Password1",
"connection": 0,
"isPasswordExpiryAllowed": true,
"title": "Demo01"
}
"@
In this case, I have changed some of the default values for my purpose with this demo user; however, the user is still created as a “Named User”. If I wanted to create the user as a “Concurrent User”, the “connection” property should be set to 1.
The user “Demo01” is created with the default password “Password1” and the user cannot change it, or is prompted to change it when the user logs on for the first time.
Next I use this REST call to create the user, and retrieve this new users ID for later user
# Post the userJson to the BI platform
$response = Invoke-RestMethod -Uri "${baseUrl}/users/user " -Method Post -Headers $headers -Body $userJson
# Retrive the user ID for later use
$userID = $response.entries.id
An example of the properties for the newly created user looks like this:
Then, I create the JSON needed for creating a new user group, since it is rather simple
# Create json for adding new group
$groupJson = @"
{
"description": "Group containing Demo Users",
"title": "Demo Users"
}
"@
It simply consist of a title and a description
Then I post the JSON and retrieve the ID for the new group for later use
# Post the groupJson to create the new group
$response = Invoke-RestMethod -Uri "${baseUrl}/userGroups/userGroup" -Method Post -Headers $headers -Body $groupJson
# Retrieve the newly created group ID
$groupId = $response.entries.id
Simple, right?
Now this part is a bit trickier. I have the userID for the newly created user and the groupID for the newly created group, now I just need to combine those.
The way I did this, was to first retrieve the object needed to add or remove users from a group
# Retrive the group object for the newly created group
$groupInfo = Invoke-RestMethod -Uri "${baseUrl}/userGroups/${groupId}" -Method Get -Headers $headers
Then I added the userID to the addMembers part of the object
# Add the new user to the group
$groupInfo.addMembers = "$userID"
Note that I added the user ID in quotes. This is because the addMembers is a string array, which means that the users you would like to add is done as a string separated with comma, like "11111,22222,33333"
Now, the ideal part for me, would just have been to post the groupInfo JSON object back, but unfortunately it contains some other derived information, that is not suited to post back. Instead I create a new object with the information from my groupInfo object, and convert the new object to JSON
# Create a new object that can be used to parse the information back
$newGroupObject = @{
cuid = $groupInfo.cuid
Description = $groupInfo.Description
addMembers = $groupInfo.addMembers
removeMembers = $groupInfo.removeMembers
id = $groupInfo.id
title = $groupInfo.title
parentID = $groupInfo.parentID
}
$newGroupJson = ConvertTo-Json($newGroupObject)
At the time of writing, I did not have any other workaround, but the complete script is on github so feel free to fork it there, and do a pull request
Now I can put back the changes, yes – do note that this command uses PUT and not POST
# Submit the changes back to the server
$response = Invoke-RestMethod -Uri "${baseUrl}/userGroups/${groupId}" -Method Put -Headers $headers -Body $newGroupJson
Write-Host $response.entries.SuccessAddingMessage
The last line writes the success response, and if everything went alright, yuu should see:
All Successfully Added
When user is created and added to the group, it should look something like this in CMC:
That is it; we have now created a user, a user group and added the user to the user group.
The last thing we could do is to delete the group and user again, if you were only testing.
In my script the lines is commented out but looks like this
<#
Invoke-RestMethod -Uri "${baseUrl}/userGroups/${groupId}" -Method Delete -Headers $headers
Invoke-RestMethod -Uri "${baseUrl}/users/$userID" -Method Delete -Headers $headers
#>
So the last thing needed is actually just so sign of, and release the token
# Log off the BI Platform (and release the logonToken)
Invoke-RestMethod -Uri $logoffURL -Method Post -Headers $headers
So that is how simple it is to create users and groups (and delete them) with the new RESTful Web Service in the upcoming Business Objects 4.2.
As mentioned, the complete script is released on GitHub, and you can find it here: https://github.com/Verakso/BO-PowerShell
Happy coding
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
46 | |
5 | |
5 | |
4 | |
3 | |
3 | |
3 | |
3 | |
2 | |
2 |