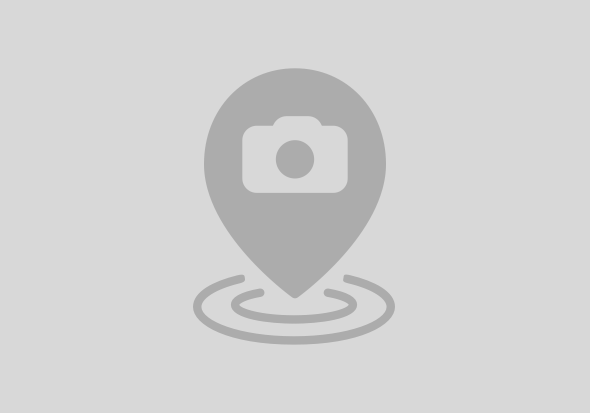
Applies to:
SAP Netweaver Master Data Management, as of MDM 7.1. For more information, visit the Master Data Management homepage.
Summary
The main purpose of this article is to provide code snippets to get started with MDM Java API, which is a very flexible way of utilizing SAP MDM features. This article provides code snippets which can be directly run in your IDE or from Command Prompt.
Author: Goutham Kanithi
Company: Mahindra Satyam
Created on: 10 June 2012
Author Bio
Goutham Kanithi has been associated with Mahindra Satyam for 18 months and has been a part of MDM practice. He is skilled in SAP MDM, Java, J2EE and completed his Bachelor’s degree in Electronics and Communication Engineering
Table of Contents
1 Business Scenario
2 Prerequisites
3 Code Snippets
3.1 To print all the running repositories in a MDM serve
3.2 To print all the tables in a repository
3.3 To print all the fields in a table.
3.4 Retrieve display field data of a table.
3.5 To retrieve selected field data from Table.
3.6 To store whole table (integer, text, lookup) data from a table in a multidimensional array.
4 Related Content
The MDM Java API is an application programming interface for the MDM software. It allows MDM customers to write customized applications for interacting with the MDM server. The MDM Java API is one of the powerful suite of MDM tools for next-generation master data management.
This article is a starting point to get started with MDM Java API. Sample code from start to end which can be directly executed.
· The MDM Java API should be downloaded from SAP Market place as shown below
· The MDM Java API libraries (JAR files) have to be referenced by the application at design time for compilation, and available at runtime for execution.
· The MDM Java API build version and MDM Server build version should match. The build version of MDM Java API can be checked as shown below
The below code retrieves the running repositories in a given MDM server
package test;
import com.sap.mdm.commands.CommandException;
import com.sap.mdm.commands.CreateServerSessionCommand;
import com.sap.mdm.commands.GetRunningRepositoryListCommand;
import com.sap.mdm.net.ConnectionException;
import com.sap.mdm.net.SimpleConnection;
import com.sap.mdm.net.SimpleConnectionFactory;
import com.sap.mdm.server.RepositoryIdentifier;
import com.sap.mdm.session.SessionException;
public class Main {
static public void main(String[] args) throws SessionException,
ConnectionException, CommandException {
String mdsName = "100.10.100.100"; // IP address or name of the Master Data Server
SimpleConnection conAccessor = SimpleConnectionFactory
.getInstance(mdsName);
// Create a user session
CreateServerSessionCommand servSessCmd = new CreateServerSessionCommand(
conAccessor);
try {
servSessCmd.execute();
} catch (CommandException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
GetRunningRepositoryListCommand repositoryList = new GetRunningRepositoryListCommand(
conAccessor);
repositoryList.execute();
RepositoryIdentifier[] repositories = repositoryList.getRepositories();
for (int w = 0; w < repositories.length; w++)
System.out.println(repositories[w].toString());
conAccessor.close();
}
}
The below code retrives the tables from a given repository
package test;
import com.sap.mdm.commands.CommandException;
import com.sap.mdm.net.ConnectionException;
import com.sap.mdm.schema.RepositorySchema;
import com.sap.mdm.schema.commands.GetRepositorySchemaCommand;
import com.sap.mdm.session.RepositorySessionContext;
import com.sap.mdm.session.SessionException;
import com.sap.mdm.session.SessionManager;
import com.sap.mdm.session.SessionTypes;
import com.sap.mdm.session.UserSessionContext;
public class Main {
static public void main(String[] args) throws SessionException,
ConnectionException, CommandException {
String mdsName = "100.10.100.100"; // IP address or name of the Master Data Server
String repositoryName = "Products"; // name of the repository
String regionName = "English [US]";
String userName = "Admin"; // enter a valid username
String password = ""; // enter the password for the above username
UserSessionContext context = new UserSessionContext(mdsName,
repositoryName, regionName, userName);
// Get an instance of the session manager
SessionManager sessionManager = SessionManager.getInstance();
// Create a user session
String ses = sessionManager.createSession(context,
SessionTypes.USER_SESSION_TYPE, password);
GetRepositorySchemaCommand grsc = new GetRepositorySchemaCommand(
(RepositorySessionContext) context);
grsc.setSession(ses);
grsc.execute();
RepositorySchema schema = grsc.getRepositorySchema();
String[] tcodes = schema.getTableCodes();
for (int i = 1; i < tcodes.length; i++) {
System.out.println("Table name is: "
+ schema.getTable(tcodes[i]).getName().get()
+ " and table code is: " + tcodes[i]);
}
// destroy the session and close the connection to the MDS
sessionManager.destroySession(context, SessionTypes.USER_SESSION_TYPE);
}
}
package test;
import com.sap.mdm.commands.CommandException;
import com.sap.mdm.net.ConnectionException;
import com.sap.mdm.schema.FieldProperties;
import com.sap.mdm.schema.RepositorySchema;
import com.sap.mdm.schema.commands.GetRepositorySchemaCommand;
import com.sap.mdm.session.RepositorySessionContext;
import com.sap.mdm.session.SessionException;
import com.sap.mdm.session.SessionManager;
import com.sap.mdm.session.SessionTypes;
import com.sap.mdm.session.UserSessionContext;
public class Main {
static public void main(String[] args) throws SessionException,
ConnectionException, CommandException {
String mdsName = "100.10.100.100"; // IP address or name of the Master Data Server
String repositoryName = "Products"; // name of the repository
String regionName = "English [US]";
String userName = "Admin"; // enter a valid username
String password = ""; // enter the password for the above username
UserSessionContext context = new UserSessionContext(mdsName,
repositoryName, regionName, userName);
// Get an instance of the session manager
SessionManager sessionManager = SessionManager.getInstance();
// Create a user session
String ses = sessionManager.createSession(context,
SessionTypes.USER_SESSION_TYPE, password);
GetRepositorySchemaCommand grsc = new GetRepositorySchemaCommand(
(RepositorySessionContext) context);
grsc.setSession(ses);
grsc.execute();
RepositorySchema schema = grsc.getRepositorySchema();
FieldProperties[] fields = schema.getFields("MDM_Products");//enter the table code
for (int i = 1; i < fields.length; i++) {
System.out.println("Field name : " + fields[i].getName().get()
+ " and Field code : " + fields[i].getCode());
}
// destroy the session and close the connection to the MDS
sessionManager.destroySession(context, SessionTypes.USER_SESSION_TYPE);
}
}
The below code retrieves the display field of each record from the main table.
package test;
import com.sap.mdm.data.Record;
import com.sap.mdm.data.RecordResultSet;
import com.sap.mdm.data.ResultDefinition;
import com.sap.mdm.data.commands.RetrieveLimitedRecordsCommand;
import com.sap.mdm.ids.TableId;
import com.sap.mdm.net.ConnectionException;
import com.sap.mdm.search.Search;
import com.sap.mdm.session.SessionException;
import com.sap.mdm.session.SessionManager;
import com.sap.mdm.session.SessionTypes;
import com.sap.mdm.session.UserSessionContext;
public class Main {
static public void main(String[] args) throws SessionException,
ConnectionException {
String mdsName = "100.10.100.100"; // IP address or name of the Master Data Server
String repositoryName = "Products"; // name of the repository
String regionName = "English [US]";
String userName = "Admin"; // enter a valid username
String password = ""; // enter the password for the above username
UserSessionContext context = new UserSessionContext(mdsName,
repositoryName, regionName, userName);
// Get an instance of the session manager
SessionManager sessionManager = SessionManager.getInstance();
// Create a user session
sessionManager.createSession(context, SessionTypes.USER_SESSION_TYPE,
password);
TableId mainTableId = new TableId(1);
ResultDefinition definition = new ResultDefinition(mainTableId);
Search search = new Search(mainTableId);
RetrieveLimitedRecordsCommand command = new RetrieveLimitedRecordsCommand(
context);
command.setResultDefinition(definition);
command.setSearch(search);
try {
command.execute();
} catch (Exception e) {
e.printStackTrace();
return;
}
RecordResultSet recordResultSet = command.getRecords();
Record[] records = recordResultSet.getRecords();
for (int i = 1; i < records.length; i++) {
System.out.println("record number " + i + " is " + records[i].getDisplayValue().toString());
}
// destroy the session and close the connection to the MDS
sessionManager.destroySession(context, SessionTypes.USER_SESSION_TYPE);
}
}
package test;
import com.sap.mdm.commands.CommandException;
import com.sap.mdm.data.Record;
import com.sap.mdm.data.RecordResultSet;
import com.sap.mdm.data.commands.RetrieveLimitedRecordsCommand;
import com.sap.mdm.extension.data.ResultDefinitionEx;
import com.sap.mdm.ids.FieldId;
import com.sap.mdm.ids.TableId;
import com.sap.mdm.net.ConnectionException;
import com.sap.mdm.schema.RepositorySchema;
import com.sap.mdm.schema.commands.GetRepositorySchemaCommand;
import com.sap.mdm.search.Search;
import com.sap.mdm.session.RepositorySessionContext;
import com.sap.mdm.session.SessionException;
import com.sap.mdm.session.SessionManager;
import com.sap.mdm.session.SessionTypes;
import com.sap.mdm.session.UserSessionContext;
public class Main {
static public void main(String[] args) throws SessionException,
ConnectionException, CommandException {
String mdsName = "100.10.100.100"; // IP address or name of the Master Data Server
String repositoryName = "Products"; // name of the repository
String regionName = "English [US]";
String userName = "Admin"; // enter a valid username
String password = ""; // enter the password for the above username
UserSessionContext context = new UserSessionContext(mdsName,
repositoryName, regionName, userName);
// Get an instance of the session manager
SessionManager sessionManager = SessionManager.getInstance();
// Create a user session
String ses = sessionManager.createSession(context,
SessionTypes.USER_SESSION_TYPE, password);
GetRepositorySchemaCommand grsc = new GetRepositorySchemaCommand(
(RepositorySessionContext) context);
grsc.setSession(ses);
grsc.execute();
RepositorySchema schema = grsc.getRepositorySchema();
String tname = "MDM_Products", selectFields[] = { "Id", "Material_Number",
"Description" };
TableId mainTableId = schema.getTableId(tname);
ResultDefinitionEx resDef = new ResultDefinitionEx("Products", context);
FieldId fid[] = new FieldId[selectFields.length];
for (int i = 0; i < selectFields.length; i++) {
fid[i] = schema.getFieldId(tname, selectFields[i]);
resDef.addSelectField(selectFields[i]);
}
resDef.setLoadAttributes(true);
Search search = new Search(mainTableId);
RetrieveLimitedRecordsCommand command = new RetrieveLimitedRecordsCommand(
context);
command.setResultDefinition(resDef);
command.setSearch(search);
command.setSession(ses);
try {
command.execute();
} catch (Exception e) {
e.printStackTrace();
}
RecordResultSet recordResultSet = command.getRecords();
Record[] records = recordResultSet.getRecords();
for (int a = 0; a < fid.length; a++) {
System.out.println("data in the field " + selectFields[a]);
for (int b = 0; b < records.length; b++) {
System.out.println("record no. " + b + "- "
+ records[b].getFieldValue(fid[a]).toString());
}
}
}
}
package test;
import com.sap.mdm.commands.CommandException;
import com.sap.mdm.data.Record;
import com.sap.mdm.data.RecordResultSet;
import com.sap.mdm.data.commands.RetrieveLimitedRecordsCommand;
import com.sap.mdm.extension.data.ResultDefinitionEx;
import com.sap.mdm.ids.FieldId;
import com.sap.mdm.ids.TableId;
import com.sap.mdm.net.ConnectionException;
import com.sap.mdm.schema.FieldProperties;
import com.sap.mdm.schema.RepositorySchema;
import com.sap.mdm.schema.commands.GetRepositorySchemaCommand;
import com.sap.mdm.search.Search;
import com.sap.mdm.session.RepositorySessionContext;
import com.sap.mdm.session.SessionException;
import com.sap.mdm.session.SessionManager;
import com.sap.mdm.session.SessionTypes;
import com.sap.mdm.session.UserSessionContext;
public class Main {
static public void main(String[] args) throws SessionException,
ConnectionException, CommandException {
String mdsName = "100.10.100.100"; // IP address or name of the Master Data Server
String repositoryName = "Products"; // name of the repository
String regionName = "English [US]";
String userName = "Admin"; // enter a valid username
String password = ""; // enter the password for the above username
UserSessionContext context = new UserSessionContext(mdsName,
repositoryName, regionName, userName);
// Get an instance of the session manager
SessionManager sessionManager = SessionManager.getInstance();
// Create a user session
String ses = sessionManager.createSession(context,
SessionTypes.USER_SESSION_TYPE, password);
GetRepositorySchemaCommand grsc = new GetRepositorySchemaCommand(
(RepositorySessionContext) context);
grsc.setSession(ses);
grsc.execute();
RepositorySchema schema = grsc.getRepositorySchema();
String tname = "MDM_Products";
int j = 0;
FieldProperties fprop[] = schema.getFields(tname);
String selectFields[] = new String[fprop.length];
for (int i = 0; i < fprop.length; i++) {
if (fprop[i].getType() <= 13) {
selectFields[j] = fprop[i].getCode();
j++;
}
}
TableId mainTableId = schema.getTableId(tname);
ResultDefinitionEx resDef = new ResultDefinitionEx("Products", context);
FieldId fid[] = new FieldId[j];
for (int i = 0; i < j; i++) {
fid[i] = schema.getFieldId(tname, selectFields[i]);
resDef.addSelectField(selectFields[i]);
}
resDef.setLoadAttributes(true);
Search search = new Search(mainTableId);
RetrieveLimitedRecordsCommand command = new RetrieveLimitedRecordsCommand(
context);
command.setResultDefinition(resDef);
command.setSearch(search);
command.setSession(ses);
try {
command.execute();
} catch (Exception e) {
e.printStackTrace();
}
RecordResultSet recordResultSet = command.getRecords();
Record[] records = recordResultSet.getRecords();
String data[][] = new String[records.length][fid.length];
for (int i = 0; i < fid.length; i++) {
System.out.println("the field is : " + selectFields[i]);
for (int g = 0; g < records.length; g++) {
try {
if (fprop[i].getType() >= 7 && fprop[i].getType() <= 10) {
try {
data[g][i] = records[g].getLookupDisplayValue(
fprop[i].getId()).toString();
} catch (NullPointerException e) {
data[g][i] = "";
}
} else
data[g][i] = records[g].getFieldValue(fprop[i].getId())
.toString();
if (data[g][i].equals("[Null]") || data[g][i].length() > 15)
data[g][i] = "";
} catch (IllegalArgumentException e) {
data[g][i] = "";
}
System.out.println(data[g][i]);
}
}
}
}
To be continued..
http://www.sap.com/platform/netweaver/components/mdm/index.epx
http://help.sap.com/saphelp_mdm71/helpdata/en/13/041975d8ce4d4287d5205816ea955a/content.htm
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
6 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |