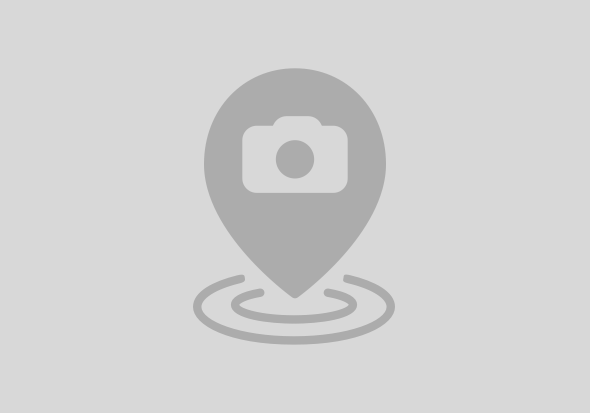
Applies To: Jdk 1.5 onwards
Author(s): Biplab Ray
Company: Tata Consultancy Services
Created on: 23rd February 2015
Author Bio:
Biplab Ray is working for Tata Consultancy Services as Assistant Consultant and development of SAP EP, composite applications using CAF, BPM, BRM, WebDynpro for Java. He also works in technologies like Java/J2EE and has depth understanding on eSOA, Webservice, Enterprise Service, XML.
Requirement:
In many applications, we might have to parse static XML files or dynamic XML object. But if we are using DOM / SAX API it would require huge code to parse the xml object.
To solve the issue, we could use JAXB API, it would not required huge code. Using JAXB API, we could convert xml object into java object and from the java object we could easily retrieve our required data. And if we required converting a java object into a XML object, via using JAXB API we could convert it, in very simple way.
Note : Few word about DOM (Document Object Model)
In the DOM approach, the parser creates a tree of objects that represents the content and organization of data in the document. In this case, the tree exists in memory. The application can then navigate through the tree to access the data it needs, and if appropriate, manipulate it.
Few Words about SAX (Simple API for XML)
Write a program that creates a SAX parser and then uses that parser to parse the XML document. The SAX parser starts at the beginning of the document. When it encounters something significant (in SAX terms, an "event") such as the start of an XML tag, or the text inside of a tag, it makes that data available to the calling application.
Few Words about JAXB (Java Architecture For XML Binding)
JAXB simplifies access to an XML document from a Java program by presenting the XML document to the program in a Java format. The first step in this process is to bind the schema for the XML document into a set of Java classes that represents the schema.
Schema: A schema is an XML specification that governs the allowable components of an XML document and the relationships between the components.
Binding: Binding a schema means generating a set of Java classes that represents the schema. All JAXB implementations provide a tool called a binding compiler to bind a schema (the way the binding compiler is invoked can be implementation-specific).
Implementation:
Step 1: Create one Data Structure like below:
Step 2: Now to implement the above structure, create two java class as below.
Root.java |
---|
/** * */ package com.example; import javax.xml.bind.annotation.XmlAccessType; import javax.xml.bind.annotation.XmlAccessorType; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; /** * @author Biplab Ray * */ @XmlRootElement(name="Root") @XmlAccessorType(XmlAccessType.FIELD) public class Root { @XmlElement(nillable=true) private String string_FirstName; @XmlElement(nillable=true) private String string_LastName; @XmlElement(nillable=true) private Child child; /** * @return the string_FirstName */ public String getString_FirstName() { return string_FirstName; } /** * @Param stringFirstName the string_FirstName to set */ public void setString_FirstName(String stringFirstName) { string_FirstName = stringFirstName; } /** * @return the string_LastName */ public String getString_LastName() { return string_LastName; } /** * @Param stringLastName the string_LastName to set */ public void setString_LastName(String stringLastName) { string_LastName = stringLastName; } /** * @return the child */ public Child getChild() { return child; } /** * @Param child the child to set */ public void setChild(Child child) { this.child = child; }
} |
The Child class as below:
Child.java |
---|
/** * */ package com.example; import javax.xml.bind.annotation.XmlAccessType; import javax.xml.bind.annotation.XmlAccessorType; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; /** * @author Biplab Ray * */ @XmlRootElement(name="Child") @XmlAccessorType(XmlAccessType.FIELD) public class Child { @XmlElement(nillable=true) private String string_StreetName; @XmlElement(nillable=true) private String string_PINCODE; @XmlElement(nillable=true) private String string_State; @XmlElement(nillable=true) private String string_Country; /** * @return the string_StreetName */ public String getString_StreetName() { return string_StreetName; } /** * @Param stringStreetName the string_StreetName to set */ public void setString_StreetName(String stringStreetName) { string_StreetName = stringStreetName; } /** * @return the string_PINCODE */ public String getString_PINCODE() { return string_PINCODE; } /** * @Param stringPINCODE the string_PINCODE to set */ public void setString_PINCODE(String stringPINCODE) { string_PINCODE = stringPINCODE; } /** * @return the string_State */ public String getString_State() { return string_State; } /** * @Param stringState the string_State to set */ public void setString_State(String stringState) { string_State = stringState; } /** * @return the string_Country */ public String getString_Country() { return string_Country; } /** * @Param stringCountry the string_Country to set */ public void setString_Country(String stringCountry) { string_Country = stringCountry; } } |
Step 3: Now create the Jaxb Util Class as below.
JaxBUtil.java |
---|
/** * */ package com.example; import java.io.StringReader; import java.io.StringWriter; import java.util.HashMap; import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBException; /** * @author Biplab Ray * */ public class JaxBUtil { static HashMap<String, JAXBContext> jaxbCache = new HashMap<String, JAXBContext>(); /** * @Param classes * @return */ private static String getJaxbContextKey(Class<?>... classes){ StringBuffer stringBuffer = new StringBuffer(); if(classes != null){ for (int i = 0; i < classes.length; i++) { String className = classes[i].getName(); ClassLoader loader = classes[i].getClassLoader(); int classLoader = 0; if(loader != null){ classLoader = loader.hashCode(); } String nextEntry = className + classLoader; stringBuffer.append(nextEntry); } }
return stringBuffer.toString(); } /** * @Param classes * @return */ private static JAXBContext getJAXBContext(Class<?>... classes){ String classKey = getJaxbContextKey(classes); JAXBContext result = jaxbCache.get(classKey);
if(result == null){ try { synchronized (JaxBUtil.class) { result = jaxbCache.get(classKey); if(result == null){ result = JAXBContext.newInstance(classes); jaxbCache.put(classKey,result); } } } catch (JAXBException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } return result; } /** * @Param object * @Param class1 * @return */ public static String jaxbMarshalConvertJavaObjectToXMLTree(Object object, Class<?> class1) { StringWriter writerPage = new StringWriter(); try { JAXBContext jaxbContextPage = getJAXBContext(class1); jaxbContextPage.createMarshaller().marshal(object, writerPage); } catch (JAXBException e) { // TODO Auto-generated catch block e.printStackTrace(); } return writerPage.toString(); } /** * This method converts XML Tree Object to Java Object. * * @Param stringReadPage - * Pass Data Stream like a String object * @Param class1 - * Pass The Data Structure Class Instance * @return - Object of Java Data Structure, you have to Cast where you * calling this method with Java Data Structure */ public static Object jaxbUNMarshalConvertXMLTreeToJavaObject( String stringReadPage, Class<?> class1) { Object dataStructureReadPage = null; try { JAXBContext jaxbContextPage = getJAXBContext(class1); dataStructureReadPage = (Object) jaxbContextPage .createUnmarshaller().unmarshal( new StringReader(stringReadPage)); } catch (JAXBException e) { // TODO Auto-generated catch block e.printStackTrace(); } return dataStructureReadPage; } } |
Step 4: Now create the test client as below:
Test.java |
---|
/** * */ package com.example; /** * @author Biplab Ray * */ public class Test { /** * @Param args */ public static void main(String[] args) { // TODO Auto-generated method stub
Root root = new Root(); root.setString_FirstName("Biplab"); root.setString_LastName("Ray"); Child child = new Child(); child.setString_Country("India"); child.setString_PINCODE("700059"); child.setString_State("West Bengal"); child.setString_StreetName("Raghnunath Pur"); root.setChild(child);
/** * Now converting Java object into XML * */ String stringXMLObject = JaxBUtil.jaxbMarshalConvertJavaObjectToXMLTree(root, Root.class);
/** * Output would be like below * <Root> * <string_FirstName>Biplab</string_FirstName> * <string_LastName>Ray</string_LastName> * <child> * <string_StreetName>Raghnunath Pur</string_StreetName> * <string_PINCODE>700059</string_PINCODE> * <string_State>West Bengal</string_State> * <string_Country>India</string_Country> * </child> * </Root> * */
System.out.println(stringXMLObject);
/** * Now Convert the XML object into Java object * And do the modification in the address parameters * And then again convert the java object into XML * */
Root rootJavaObject = (Root)JaxBUtil.jaxbUNMarshalConvertXMLTreeToJavaObject(stringXMLObject, Root.class);
Child child2 = rootJavaObject.getChild(); child2.setString_PINCODE("700156"); rootJavaObject.setChild(child2);
String string_AfterModificationOfAddressPinCode = JaxBUtil.jaxbMarshalConvertJavaObjectToXMLTree(rootJavaObject, Root.class);
/** * Output would be like below * <Root> * <string_FirstName>Biplab</string_FirstName> * <string_LastName>Ray</string_LastName> * <child> * <string_StreetName>Raghnunath Pur</string_StreetName> * <string_PINCODE>700156</string_PINCODE> * <string_State>West Bengal</string_State> * <string_Country>India</string_Country> * </child> * </Root> * */
System.out.println(string_AfterModificationOfAddressPinCode);
} } |
So, with help of JAXB without any parsing logic we could easily retrieve the data from xml structure and if required we could easily modify the value of xml.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |