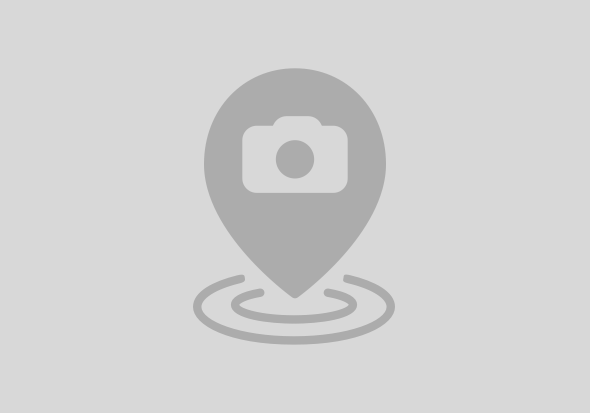
Applies To : JDK 1.5 onwards
Author(s): Biplab Ray
Company: Tata Consultancy Services
Created on: 25th February 2015
Author Bio :
Biplab Ray is working for Tata Consultancy Services as Assistant Consultant and development of SAP EP , composite applications using CAF, BPM, BRM, WebDynpro for Java. He also works in technologies like Java/J2EE and has depth understanding on eSOA, Webservice, Enterprise Service, XML.
Requirement :
In many situation, we might required to fetch few values from XML object. But to retrieve the value we have to write the parsing code.
But if we use the XPath, then with help of XPath expression , easily we could retrieve our desired value from XML.
Few Word About XPath:
XPath uses language syntax much similar to what we already know. The syntax is a mix of basic programming language expressions (wild cards such as $x*6) and Unix-like path expressions (such as /inventory/author). In addition to the basic syntax, XPath provides a set of useful functions (such as count() or contains(), much similar to utility functions calls) that allow us to search for various data fragments inside the document.
Selecting nodes
Expression | Description |
---|---|
nodename | Selects all nodes with the name “nodename“ |
/ | Selects from the root node |
// | Selects nodes in the document from the current node that match the selection no matter where they are |
. | Selects the current node |
.. | Selects the parent of the current node |
@ | Selects attributes |
Implementation:
Below imports required :
import java.io.StringReader;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpression;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.xml.sax.InputSource;
Write the below method.
/**
* This method returns a String object, when we required to get a single
* value form an XML Tree string object, we should use this method.
*
* @Param stringCompile -
* Pass the Compile String object for Searching in the XML Tree
* Object
* @Param stringSource -
* Pass the XML Tree object as String
* @return - Return the Expected value as String object
*/
public static String getXPathValue(String stringCompile, String stringSource) {
StringBuilder stringBuilder = new StringBuilder();
XPathFactory pathFactory = XPathFactory.newInstance();
XPath path = pathFactory.newXPath();
try {
XPathExpression pathExpression = path.compile(stringCompile);
Object result = pathExpression.evaluate(new InputSource(
new StringReader(stringSource)), XPathConstants.STRING);
if (result instanceof String) {
stringBuilder.append(result.toString());
}
} catch (XPathExpressionException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return stringBuilder.toString();
}
And from the client ( main method of java class) or invoked method we should pass the input parameters as below.
String stringSource =
"<?xml version=\"1.0\" encoding=\"UTF-8\" standalone=\"yes\"?>" +
"<Root><string_FirstName>Biplab</string_FirstName>" +
"<string_LastName>Ray</string_LastName>" +
"<child><string_StreetName>Raghnunath Pur</string_StreetName>" +
"<string_PINCODE>700156</string_PINCODE><string_State>West Bengal</string_State>" +
"<string_Country>India</string_Country></child></Root>";
/*Below XPath expression, would retrieve the value of PIN code : It would return 700156*/
String stringCompile = "//string_PINCODE/text()";
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
8 | |
7 | |
5 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |