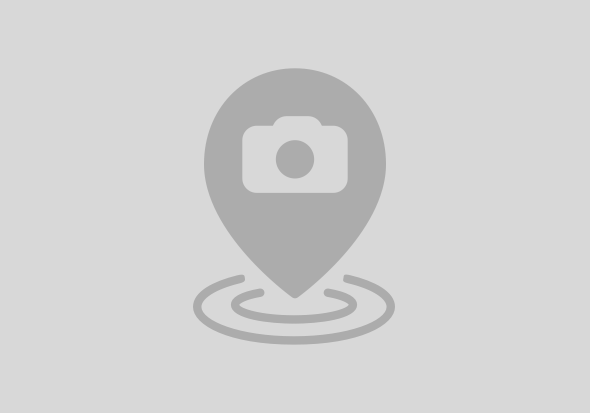
<!DOCTYPE html>
<html>
<head>
<!--<meta http-equiv="Content-Security-Policy" content="default-src 'self' http://localhost:8080 'unsafe-inline' data: gap: https://ssl.gstatic.com 'unsafe-eval'; style-src 'self' 'unsafe-inline'; media-src *">-->
<script>
window.onerror = onError;
var appCID = window.localStorage.getItem("appcid");
var connectionData = {
DeviceType : "Windows" // Windows, iOS, Android etc
};
var settingsData = {
DevicePhoneNumber : "123 456 7899" // one of the properties listed as sup:ReadOnly="false"
};
var userName = "i82XXXX";
var password = "XXXXXXX";
var host = "localhost"; //note if this value changes, also change Content Security Policy (the meta tag on line 4)
var baseAppIDStr = "/odata/applications/latest/com.mycompany.logon/";
function onError(msg, url, line) {
var idx = url.lastIndexOf("/");
var file = "unknown";
if (idx > -1) {
file = url.substring(idx + 1);
}
alert("An error occurred in " + file + " (at line # " + line + "): " + msg);
return false; //suppressErrorAlert;
}
function onLoad() {
var userEl = document.getElementById("user");
var passwordEl = document.getElementById("password");
var hostEl = document.getElementById("host");
userEl.value = userName;
passwordEl.value = password;
hostEl.value = host;
}
function getSMPURL() {
return "http://" + document.getElementById("host").value + ":8080";
}
function getUserName() {
return document.getElementById("user").value;
}
function getPassword() {
return document.getElementById("password").value;
}
function register() {
if (appCID) {
alert("Already Registered");
return;
}
sUrl = getSMPURL() + baseAppIDStr + "Connections";
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
console.log(xmlhttp.responseText);
if (xmlhttp.readyState == 4) {
if (xmlhttp.status == 201) {
var startStr = "ApplicationConnectionId>";
var start = xmlhttp.responseText.indexOf(startStr);
if (start != -1) {
var end = xmlhttp.responseText.indexOf("</d:ApplicationConnectionId");
appCID = xmlhttp.responseText.substring(start + startStr.length, end);
window.localStorage.setItem("appcid", appCID);
alert("Registered Successfully");
}
}
else {
alert("Registration failed with a result code of " + xmlhttp.status);
}
}
}
xmlhttp.open("POST", sUrl, true);
xmlhttp.setRequestHeader("Authorization", "Basic " + btoa(getUserName() + ":" + getPassword()));
xmlhttp.setRequestHeader("Accept", "application/atom+xml");
xmlhttp.setRequestHeader("Content-Type", "application/json");
xmlhttp.send(JSON.stringify(connectionData));
}
function unRegister() {
if (!appCID) {
alert("Not Registered");
return;
}
sUrl = getSMPURL() + baseAppIDStr + "Connections('" + appCID + "')";
appCID = "";
window.localStorage.setItem("appcid", "");
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
alert("Successfully Unregistered");
}
}
xmlhttp.open("DELETE", sUrl, true);
xmlhttp.setRequestHeader("Authorization", "Basic " + btoa(getUserName() + ":" + getPassword()));
xmlhttp.send();
}
function getSettingsMetaData() {
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
console.log(xmlhttp.responseText);
alert("Check the WebInsepctor console for the settings metadata details or the Network > Preview tab.");
}
}
sUrl = getSMPURL() + baseAppIDStr + "$metadata";
xmlhttp.open("GET", sUrl, true);
xmlhttp.setRequestHeader("Authorization", "Basic " + btoa(getUserName() + ":" + getPassword()));
xmlhttp.setRequestHeader("Accept", "application/xml"); //setting this so it is easier to view response in Network > Preview tab.
xmlhttp.send();
}
function getSettings() {
if (!appCID) {
alert("Must be registered");
return;
}
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
console.log(xmlhttp.responseText);
alert("Check the WebInsepctor console for the settings details or the Network > Preview tab.");
}
}
sUrl = getSMPURL() + baseAppIDStr +"Connections('" + appCID + "')";
xmlhttp.open("GET", sUrl, true);
xmlhttp.setRequestHeader("Authorization", "Basic " + btoa(getUserName() + ":" + getPassword()));
xmlhttp.setRequestHeader("Accept", "application/json"); //setting this so it is easier to view response in Network > Preview tab.
xmlhttp.send();
}
function updateSettings() {
if (!appCID) {
alert("Must be registered");
return;
}
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
console.log(xmlhttp.responseText);
alert("Setting DevicePhoneNumber updated. Call GetSettings to see updated value");
}
}
sUrl = getSMPURL() + baseAppIDStr + "Connections('" + appCID + "')";
xmlhttp.open("POST", sUrl, true);
xmlhttp.setRequestHeader("Authorization", "Basic " + btoa(getUserName() + ":" + getPassword()));
xmlhttp.setRequestHeader("X-HTTP-METHOD", "MERGE");
xmlhttp.setRequestHeader("Content-Type", "application/json");
xmlhttp.send(JSON.stringify(settingsData));
}
</script>
</head>
<body onload="onLoad()">
<h1>Settings Exchange Sample</h1>
SMP 3.0 Host: <input type="text" id="host" value=""><br>
User ID: <input type="text" id="user" value=""><br>
Password: <input type="text" id="password" value=""><br>
<button id="register" onclick="register()">Register</button>
<button id="unregister" onclick="unRegister()">Unregister</button>
<button id="getsettingsMetaData" onclick="getSettingsMetaData()">Get Settings MetaData</button>
<button id="getsettings" onclick="getSettings()">Get Settings</button>
<button id="updateSettings" onclick="updateSettings()">Update Settings</button>
</body>
</html>
cd C:\Kapsel_Projects\LoggerDemo
cd ~/Documents/Kapsel_Projects/LoggerDemo
cordova plugin add kapsel-plugin-settings --searchpath %KAPSEL_HOME%/plugins
or
cordova plugin add kapsel-plugin-settings --searchpath $KAPSEL_HOME/plugins
cordova prepare
LoggerDemo/plugins/kapsel-plugin-settings/www/settings.js
getLogLevel(); //updates the Log Level select to the value returned to the Kapsel app from the SMP 3.0 server
cordova run android
or
cordova run ios
www/plugins/kapsel-plugin-logger/www/logger.js
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
18 | |
12 | |
11 | |
8 | |
8 | |
7 | |
6 | |
6 | |
6 | |
5 |