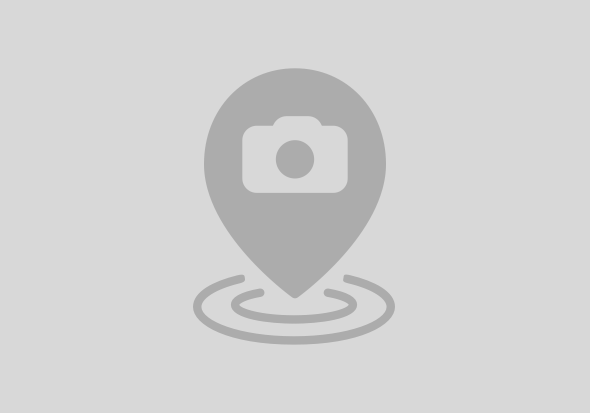
I know late again, sorry. My excuse this time? I've just bought a Garmin Edge 305 GPS for my bike and I seem to have become obsessed with riding, my bike that is! Now down to business, last time we wrote our first test script this time we're going to use the Ruby unit testing framework to run the script.
OUR FIRST TEST CASE
First create a new file called c:\watir\scripts\myfirsttc.rb with the following content. I know this code does not look particularly good in HTML but should be OK to cut and paste into an editor. Note to self: Find out which tags to use for posting code to your SAP blog!
require 'watir'
require 'test/unit'
class MyFirstTC < Test::Unit::TestCase
def setup
@ie = Watir::IE.new
end
def test_login
@ie.goto('http://sweet:50000/irj/portal')
@ie.text_field(:id, 'logonuidfield').set('myuserid')
@ie.text_field(:id, 'logonpassfield').set('mypassword')
@ie.button(:name, 'uidPasswordLogon').click
assert(@ie.contains_text('Bahh I failed!'), 'Text not found!')
end
end
def teardown
@ie.close
end
Now lets just break this script down and explain the new bits.
REFERENCE THE TEST LIBRARY
We need to reference the Ruby Unit Test Library this is done with one simple line, nothing new here.
require 'test/unit'
THE TEST CASE CLASS
To use the unit test framework our class needs to inherit from the Test::Unit::TestCase base class. This is done using the Ruby operator '<'.
class MyFirstTC < Test::Unit::TestCase
THE SETUP METHOD
Before each test method the setup method automatically runs, this allows you to do any required setup before a test method is run. The setup method runs before EVERY test method so if your test case class contained 100 test methods the setup method would be run 100 times. In our case we simply create a class level variable called @ie which will be available to our test method.
NOTE: The @ symbol is significant as this is how Ruby defines class level variables.
def setup
@ie = Watir::IE.new
end
THE TEST METHOD
Now this is were our actual testing goes on. One thing to note about our test method is it must start with the prefix 'test_' this simple naming convention is how the test framework knows which methods are test methods and which are not. So we call out login test 'test_login'.
def test_login
@ie.goto('http://sweet:50000/irj/portal')
@ie.text_field(:id, 'logonuidfield').set('myuserid')
@ie.text_field(:id, 'logonpassfield').set('mypassword')
@ie.button(:name, 'uidPasswordLogon').click
assert(@ie.contains_text('Bahh I failed!'), 'Text not found!')
end
assert(@ie.contains_text('Bahh I failed!'), 'Text not found!')
The assert method checks that the first parameter equals true, if not it raises and error and displays the descriptive message. So this line is actually saying if you cannot find the text 'Bahh I failed!' then raise an error and display the message 'Text not found!'. Now I know we're not going to find the text 'Bahh I failed!' during this test but to be sure the test is working we MUST first make it fail. Also I want you to see what happens when we get a failed assert.
NOTE: The Ruby unit testing framework has a lots of different assertion methods which you will want to checkout.
THE TEARDOWN METHOD
The opposite of the setup method the teardown method is called automatically after each and every test method. In our case we simply close Internet Explorer.
def teardown
@ie.close
end
THE RUN
Now that we know what the script is doing lets run our test. Open a DOS window and type the following commands.
cd c:\watir\scripts
ruby myfirsttc.rb
c:\watir\scripts>
ruby myfirsttc.rbLoaded suite c:/watir/scripts/myfirsttc
Started
F
Finished in 5.609 seconds.
1) Failure:
test_login(MyFirstTC) c:/watir/scripts/myfirsttc.rb:20:
Text not found!.
THE REPORT
Although it does not look like much and there is no fancy GUI do not be deceived there is quite a bit of information here. First it tells us how long the tests take to run.
Finished in 5.609 seconds.
1) Failure:
test_login(MyFirstTC) c:/watir/scripts/myfirsttc.rb:*20*:
We can also see the descriptive message we used in our code and also the actual Watir error message.
Text not found!.
NOTE: A test method can contain as many assertions as you like. In fact it is usual that a test method will contain several assertions, they are after all the core of your tests.
FIXING THE TEST
Ok we've seen a failing test so lets go ahead and fix it. We simply need to change the assert line to look for a different piece of text. Lets look for 'Log Off' as we should see this text if our log on was successfull right?
assert(@ie.contains_text('Log Off'), 'Text not found!')
Run the test again using THE RUN procedure above. You should now have a passing test. Congratulations!
Started
.
Finished in 5.359 seconds.
1 tests, 1 assertions, 0 failures, 0 errors
HUNDREDS OF TESTS
So that's one test but how do you run hundreds of tests? Simple add more test methods to your test case class. When you run the test case the framework will pickup your new test method and run that too. I tend to have one test case class for each module I am testing and add new test methods as I go. Eventually you will end up with hundreds of tests and the total confidence in your code which comes along with it.
COMING UP IN PART FIVE
That's quite big post (for me anyway!) so I'll leave it there until next time when we'll go through my top tips which should help with your testing. That is providing I can stay off my bike for long enough to write it!